PL/SQL is Oracle's Procedural Language extension to SQL. It is commonly used to write data-centric programs to manipulate data in an Oracle database. Here's a basic primer to help beginners get started.
Chapter Objectives
In this Chapter, you will learn about:
*
The Nature of a Computer Program and Programming Languages
*
Good Programming Practices
Computers play a large role in the modern world. No doubt you realize how crucial they have become to running any business today; they have also become one of the sources of entertainment in our lives. You probably use computers for your everyday tasks as well, such as sending e-mail, paying bills, shopping, reading the latest news on the Internet, or even playing games.
A computer is a sophisticated device. However, it is important to remember that it is still only a device and cannot think on its own. In order to be useful, a computer needs instructions to follow. Facilities such as programming languages allow programmers to provide computers with a list of instructions called programs. These programs tell a computer what actions to perform. As a result, programming languages and computer programs play an important role in today's technology.
Lab 1.1 The Nature of a Computer Program and Programming Languages
Lab Objectives
After this Lab, you will be able to:
*
Understand the Nature of Computer Programs and Programming Languages
*
Understand the Differences between Interpreted and Compiled Languages
A computer needs instructions to follow because it cannot think on its own. For instance, when playing a game of solitaire you must choose which card to move. Each time a card is moved, a set of instructions has been executed to carry out the move. These instructions compose only a small part of the solitaire program. This program comprises many more instructions that allow a user to perform actions, such as beginning or ending a game, selecting a card's color, and so forth. Therefore, a computer program comprises instructions that direct the actions of the computer. In essence, a program plays the role of guide for a computer. It tells the computer what steps in what order should be taken to complete a certain task successfully.
Computer programs are created with the help of programming languages. A programming language is a set of instructions consisting of rules, syntax, numerical and logical operators, and utility functions. Programmers can use programming languages to create a computer program. There are many different programming languages available today. However, all programming languages can be divided into three major groups: machine languages, assembly languages, and high-level languages.
TIP
Words such as statement or command are often used when talking about instructions issued by a program to a computer. These terms are interchangeable.
Machine Languages
Machine language is the native language of a particular computer because it is defined by the hardware of the computer. Each instruction or command is a collection of zeros and ones. As a result, machine language is the hardest language for a person to understand, but it is the only language understood by the computer. All other programming languages must be translated into machine language. Consider the following example of the commands issued in the machine language.
For Example
Consider the mathematical notation X = X + 1. In programming, this notation reads the value of the variable is incremented by one. In the following example, you are incrementing the value of the variable by 1 using machine language specific to an Intel processor.
1010 0001 1110 0110 0000 0001
0000 0011 0000 0110 0000 0001 0000 0000
1010 0011 1110 0110 0000 0001
Assembly Languages
Assembly language uses English-like abbreviations to represent operations performed on the data. A computer cannot understand assembly language directly. A program written in assembly language must be translated into machine language with the help of the special program called an assembler. Consider the following example of the commands issued in assembly language.
For Example
In this example, you are increasing the value of the variable by 1 as well. This example is also specific to an Intel processor.
MOV AX, [01E6]
ADD AX, 0001
MOV [01E6], AX
High-Level Languages
A high-level language uses English-like instructions and common mathematical notations. High-level languages allow programmers to perform complicated calculations with a single instruction. However, it is easier to read and understand than machine and assembly languages, and it is not as time-consuming to create a program in high-level language as it is in machine or assembly language.
For Example
variable := variable + 1;
This example shows the simple mathematical operation of addition. This instruction can be easily understood by anyone without programming experience and with basic mathematical knowledge.
Differences between Interpreted and Compiled Languages
High-level languages can be divided into two groups: interpreted and compiled. Interpreted languages are translated into machine language with the help of another program called an interpreter. The interpreter translates each statement in the program into machine language and executes it immediately before the next statement is examined.
A compiled language is translated into machine language with the help of the program called a compiler. Compilers translate English-like statements into machine language. However, all of the statements must be translated before a program can be executed. The compiled version of the program is sometimes referred to as an executable.
An interpreted program must be translated into machine language every time it is run. A compiled program is translated into machine language only once when it is compiled. The compiled version of the program can then be executed as many times as needed.
SOURCE:http://www.informit.com/articles/article.aspx?p=30663
Chapter Objectives
In this Chapter, you will learn about:
*
The Nature of a Computer Program and Programming Languages
*
Good Programming Practices
Computers play a large role in the modern world. No doubt you realize how crucial they have become to running any business today; they have also become one of the sources of entertainment in our lives. You probably use computers for your everyday tasks as well, such as sending e-mail, paying bills, shopping, reading the latest news on the Internet, or even playing games.
A computer is a sophisticated device. However, it is important to remember that it is still only a device and cannot think on its own. In order to be useful, a computer needs instructions to follow. Facilities such as programming languages allow programmers to provide computers with a list of instructions called programs. These programs tell a computer what actions to perform. As a result, programming languages and computer programs play an important role in today's technology.
Lab 1.1 The Nature of a Computer Program and Programming Languages
Lab Objectives
After this Lab, you will be able to:
*
Understand the Nature of Computer Programs and Programming Languages
*
Understand the Differences between Interpreted and Compiled Languages
A computer needs instructions to follow because it cannot think on its own. For instance, when playing a game of solitaire you must choose which card to move. Each time a card is moved, a set of instructions has been executed to carry out the move. These instructions compose only a small part of the solitaire program. This program comprises many more instructions that allow a user to perform actions, such as beginning or ending a game, selecting a card's color, and so forth. Therefore, a computer program comprises instructions that direct the actions of the computer. In essence, a program plays the role of guide for a computer. It tells the computer what steps in what order should be taken to complete a certain task successfully.
Computer programs are created with the help of programming languages. A programming language is a set of instructions consisting of rules, syntax, numerical and logical operators, and utility functions. Programmers can use programming languages to create a computer program. There are many different programming languages available today. However, all programming languages can be divided into three major groups: machine languages, assembly languages, and high-level languages.
TIP
Words such as statement or command are often used when talking about instructions issued by a program to a computer. These terms are interchangeable.
Machine Languages
Machine language is the native language of a particular computer because it is defined by the hardware of the computer. Each instruction or command is a collection of zeros and ones. As a result, machine language is the hardest language for a person to understand, but it is the only language understood by the computer. All other programming languages must be translated into machine language. Consider the following example of the commands issued in the machine language.
For Example
Consider the mathematical notation X = X + 1. In programming, this notation reads the value of the variable is incremented by one. In the following example, you are incrementing the value of the variable by 1 using machine language specific to an Intel processor.
1010 0001 1110 0110 0000 0001
0000 0011 0000 0110 0000 0001 0000 0000
1010 0011 1110 0110 0000 0001
Assembly Languages
Assembly language uses English-like abbreviations to represent operations performed on the data. A computer cannot understand assembly language directly. A program written in assembly language must be translated into machine language with the help of the special program called an assembler. Consider the following example of the commands issued in assembly language.
For Example
In this example, you are increasing the value of the variable by 1 as well. This example is also specific to an Intel processor.
MOV AX, [01E6]
ADD AX, 0001
MOV [01E6], AX
High-Level Languages
A high-level language uses English-like instructions and common mathematical notations. High-level languages allow programmers to perform complicated calculations with a single instruction. However, it is easier to read and understand than machine and assembly languages, and it is not as time-consuming to create a program in high-level language as it is in machine or assembly language.
For Example
variable := variable + 1;
This example shows the simple mathematical operation of addition. This instruction can be easily understood by anyone without programming experience and with basic mathematical knowledge.
Differences between Interpreted and Compiled Languages
High-level languages can be divided into two groups: interpreted and compiled. Interpreted languages are translated into machine language with the help of another program called an interpreter. The interpreter translates each statement in the program into machine language and executes it immediately before the next statement is examined.
A compiled language is translated into machine language with the help of the program called a compiler. Compilers translate English-like statements into machine language. However, all of the statements must be translated before a program can be executed. The compiled version of the program is sometimes referred to as an executable.
An interpreted program must be translated into machine language every time it is run. A compiled program is translated into machine language only once when it is compiled. The compiled version of the program can then be executed as many times as needed.
SOURCE:http://www.informit.com/articles/article.aspx?p=30663
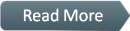